This post has been moved to http://srinichekuri.com/2016/01/09/select2-jquery-plugin/
**********************************************************************
Select2 is famous Jquery plugin that allows customization of select component with support for searching, tagging, remote data sets, infinite scrolling etc.
I had a chance to work on select2 plugin off late and I was able to use it with using various options. I faced some serious issues while working on these options and realized that there were lot of developers who inquired on web on how to use select2 in these scenarios but there are no proper responses/documentation with examples for these scenarios.
Below are the various examples for select2 that I have published.
Select2 3.5.2 version was used for below examples. Be advised to read through specs before you process any further.
Below are Select2 Parameters that I have used for examples :
- minimumInputLength: minimum number of characters before starting a search. Eg: 2.
- minimumResultsForSearch: limit on the results to be displayed.
- placeHolder: Place holder to be shown when there are no selections made. Eg: ‘Select Employee’
- tokenSeparators: Character set that can be used as delimeters to enter multiple values. Eg: [‘,’]
- Multiple: Boolean value to indicate if multiple values can be selected
- Id: function used to get id from the object that is selected
- Ajax: Option to be used for built in ajax query selection.
- url: url to make ajax call.
- datatype: data type of request. Eg: ‘json’
- params: Extra paramters to be passed on in request. Eg: ‘{contentType: “application/json”}’
- data: query parameters for ajax request
- results: builds query result object from ajax response.
- formatSelection: format the selection that is shown.
- formatResult: Function used to render a result that is shown to user for selection. Eg: results that are shown in dropdown.
Select2 – Tags (Click Here for working code):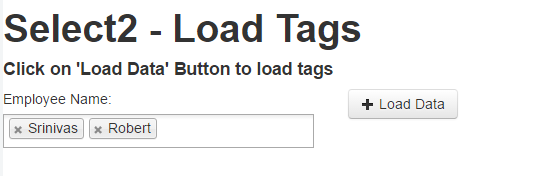
<div id="maincontainer" class="clearfix">
<!-- main content s-->
<div id="contentwrapper">
<div class="main_content">
<div class="row-fluid">
<div class="span6" id="employeeColumn">
<div class="control-group">
<label class="control-label">Employee Name: </label>
<div class="controls">
<input type="text" class="tags" id="select2_tags_id" />
</div>
</div>
<!--control group -->
</div>
<!-- Employee Column -->
<button id="loadDataButton" class="btn" type="submit"><i class="icon-plus" alt="OK"></i> Load Data </button>
</div>
<!-- row-fluid -->
</div>
<!-- main_content -->
</div>
<!-- content wrapper -->
</div>
Java script for Select2 Tags.
$(document).ready(function() {
$('#select2_tags_id').select2({
tokenSeparators: [','],
tags: true,
maximumSelectionSize: 10,
minimumResultsForSearch: Infinity,
multiple: true,
dropdownCss: {
display: 'none'
}
});
$('#loadDataButton').on('click', function() {
$('#select2_tags_id').select2('val', ['Srinivas', 'Robert']);
});
});
Select2 – Ajax – Single Selection (Click Here for working code):
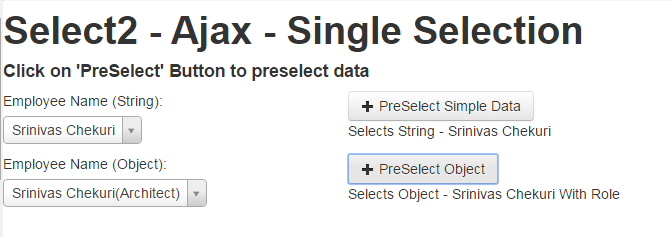
Below code is for dynamic search using Ajax and for single selection.
<div id="maincontainer" class="clearfix">
<!-- main content s-->
<div id="contentwrapper">
<div class="main_content">
<div class="row-fluid">
<div class="span6" id="employeeColumn">
<div class="control-group">
<label class="control-label">Employee Name (String): </label>
<div class="controls">
<input type="text" id="select2_ajax_simple_id" />
</div>
</div>
<!--control group -->
</div>
<!-- Employee Column -->
<button id="preselectSimpleDataButton" class="btn" type="submit"><i class="icon-plus" alt="OK"></i> PreSelect Simple Data </button>
<label>Selects String - Srinivas Chekuri </label>
</div>
<!-- row-fluid -->
<!--Code for Select2 Object -->
<div class="row-fluid">
<div class="span6" id="employeeComplexColumn">
<div class="control-group">
<label class="control-label">Employee Name (Object): </label>
<div class="controls">
<input type="text" id="select2_ajax_complex_id" />
</div>
</div>
<!--control group -->
</div>
<!-- Employee Column -->
<button id="preselectObjectDataButton" class="btn" type="submit"><i class="icon-plus" alt="OK"></i> PreSelect Object</button>
<label>Selects Object - Srinivas Chekuri With Role </label>
</div>
<!-- row-fluid -->
</div>
<!-- main_content -->
</div>
<!-- content wrapper -->
</div>
Java script for Select2 configuration and selection for simple data (String).
$('#select2_ajax_simple_id').select2({
minimumInputLength: 1,
placeholder: &amp;amp;amp;amp;amp;quot;Search Employee&amp;amp;amp;amp;amp;quot;,
//data:o,
id: function(i) {
return i;
},
initSelection: function(element, callback) {
},
ajax: {
type: 'post',
url: &amp;amp;amp;amp;amp;quot;/echo/json/&amp;amp;amp;amp;amp;quot;,
allowClear: true,
dataType: 'json',
delay: 250,
params: {
contentType: &amp;amp;amp;amp;amp;quot;application/json&amp;amp;amp;amp;amp;quot;
},
data: function(term, page) {
//Code for dummy ajax response
return {
json: simple_employee_response,
delay: 0
};
},
results: function(data, page) {
return {
results: data
};
},
cache: false
},
formatResult: function(i) {
return ' &amp;amp;amp;amp;amp;lt; div &amp;amp;amp;amp;amp;gt; '+i+' &amp;amp;amp;amp;amp;lt; /div&amp;amp;amp;amp;amp;gt;
'; }, // Formats results in drop down
formatSelection: function(i) {
return ' &amp;amp;amp;amp;amp;lt; div &amp;amp;amp;amp;amp;gt; '+i+' &amp;amp;amp;amp;amp;lt; /div&amp;amp;amp;amp;amp;gt;
'; }, //Formats result that is selected
dropdownCssClass: &amp;amp;amp;amp;amp;quot;bigdrop&amp;amp;amp;amp;amp;quot;, // apply css that makes the dropdown taller
escapeMarkup: function(m) {
return m;
} // we do not want to escape markup since we are displaying html in results
});
Java script for Select2 configuration and selection based on Objects.
Note: Html is the same as shown above.
$('#select2_ajax_complex_id').select2({
minimumInputLength: 1,
placeholder: &amp;amp;amp;amp;amp;quot;Search Employee&amp;amp;amp;amp;amp;quot;,
//data:o,
id: function(i) {
return i;
},
initSelection: function(element, callback) {
},
ajax: {
type: 'post',
url: &amp;amp;amp;amp;amp;quot;/echo/json/&amp;amp;amp;amp;amp;quot;,
allowClear: true,
dataType: 'json',
delay: 250,
params: {
contentType: &amp;amp;amp;amp;amp;quot;application/json&amp;amp;amp;amp;amp;quot;
},
data: function(term, page) {
//Code for dummy ajax response
return {
json: complex_employee_response,
delay: 0
};
},
results: function(data, page) {
return {
results: data
};
},
cache: false
},
formatResult: function(i) {
return ' &amp;amp;amp;amp;amp;lt; div &amp;amp;amp;amp;amp;gt; '+i.name+' ('+i.role+')
'+' &amp;amp;amp;amp;amp;lt; /div&amp;amp;amp;amp;amp;gt;
'; }, // Formats results in drop down
formatSelection: function(i) {
return ' &amp;amp;amp;amp;amp;lt; div &amp;amp;amp;amp;amp;gt; '+i.name+' ('+i.role+')
'+' &amp;amp;amp;amp;amp;lt; /div&amp;amp;amp;amp;amp;gt;
'; }, //Formats result that is selected
dropdownCssClass: &amp;amp;amp;amp;amp;quot;bigdrop&amp;amp;amp;amp;amp;quot;, // apply css that makes the dropdown taller
escapeMarkup: function(m) {
return m;
} // we do not want to escape markup since we are displaying html in results
})
$('#preselectSimpleDataButton').on('click', function() {
// alert('I am here');
$('#select2_ajax_simple_id').select2('data', 'Srinivas Chekuri');
});
$('#preselectObjectDataButton').on('click', function() {
// alert('I am here');
var o = new Object;
o.id = &amp;amp;amp;amp;amp;quot;1&amp;amp;amp;amp;amp;quot;;
o.name = &amp;amp;amp;amp;amp;quot;Srinivas Chekuri&amp;amp;amp;amp;amp;quot;;
o.role = &amp;amp;amp;amp;amp;quot;Architect&amp;amp;amp;amp;amp;quot;;
$('#select2_ajax_complex_id').select2('data', o);
});
Select2 – Ajax – Single Selection (Click Here for working code):
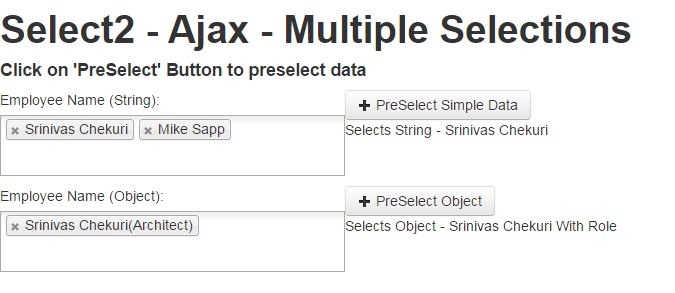
Below code is for dynamic search using Ajax and to have multiple selections.
<div id="maincontainer" class="clearfix">
<!-- main content s-->
<div id="contentwrapper">
<div class="main_content">
<div class="row-fluid">
<div class="span6" id="employeeColumn">
<div class="control-group">
<label class="control-label">Employee Name (String): </label>
<div class="controls">
<input type="text" class="tags" id="select2_ajax_simple_id" />
</div>
</div>
<!--control group -->
</div>
<!-- Employee Column -->
<button id="preselectSimpleDataButton" class="btn" type="submit"><i class="icon-plus" alt="OK"></i> PreSelect Simple Data </button>
<label>Selects String - Srinivas Chekuri </label>
</div>
<!-- row-fluid -->
<!--Code for Select2 Object -->
<div class="row-fluid">
<div class="span6" id="employeeComplexColumn">
<div class="control-group">
<label class="control-label">Employee Name (Object): </label>
<div class="controls">
<input type="text" class="tags" id="select2_ajax_complex_id" />
</div>
</div>
<!--control group -->
</div>
<!-- Employee Column -->
<button id="preselectObjectDataButton" class="btn" type="submit"><i class="icon-plus" alt="OK"></i> PreSelect Object</button>
<label>Selects Object - Srinivas Chekuri With Role </label>
</div>
<!-- row-fluid -->
</div>
<!-- main_content -->
</div>
<!-- content wrapper -->
</div>
Java script for Select2 configuration and selection for simple data (Strings) with multiple selections.
$('#select2_ajax_simple_id').select2({
tags: true,
maximumSelectionSize: 10,
minimumResultsForSearch: Infinity,
multiple: true,
minimumInputLength: 1,
placeholder: &amp;amp;amp;amp;amp;quot;Search Employee&amp;amp;amp;amp;amp;quot;,
//data:o,
id: function(i) {
return i;
},
initSelection: function(element, callback) {
},
ajax: {
type: 'post',
url: &amp;amp;amp;amp;amp;quot;/echo/json/&amp;amp;amp;amp;amp;quot;,
allowClear: true,
dataType: 'json',
delay: 250,
params: {
contentType: &amp;amp;amp;amp;amp;quot;application/json&amp;amp;amp;amp;amp;quot;
},
data: function(term, page) {
//Code for dummy ajax response
return {
json: simple_employee_response,
delay: 0
};
},
results: function(data, page) {
return {
results: data
};
},
cache: false
},
formatResult: function(i) {
return ' &amp;amp;amp;amp;amp;lt; div &amp;amp;amp;amp;amp;gt; ' + i + ' &amp;amp;amp;amp;amp;lt; /div&amp;amp;amp;amp;amp;gt;
';
}, // Formats results in drop down
formatSelection: function(i) {
return ' &amp;amp;amp;amp;amp;lt; div &amp;amp;amp;amp;amp;gt; ' + i + ' &amp;amp;amp;amp;amp;lt; /div&amp;amp;amp;amp;amp;gt;
';
}, //Formats result that is selected
dropdownCssClass: &amp;amp;amp;amp;amp;quot;bigdrop&amp;amp;amp;amp;amp;quot;, // apply css that makes the dropdown taller
escapeMarkup: function(m) {
return m;
} // we do not want to escape markup since we are displaying html in results
});
$('#preselectSimpleDataButton').on('click', function() {
$('#select2_ajax_simple_id').select2('data', ['Srinivas Chekuri']);
});
Java script for Select2 configuration and selection based on Objects.
Note: Html is the same as shown above.
$('#select2_ajax_complex_id').select2({
tags: true,
maximumSelectionSize: 10,
minimumResultsForSearch: Infinity,
multiple: true,
minimumInputLength: 1,
placeholder: &amp;amp;amp;amp;amp;quot;Search Employee&amp;amp;amp;amp;amp;quot;,
//data:o,
id: function(i) {
return i;
},
initSelection: function(element, callback) {
},
ajax: {
type: 'post',
url: &amp;amp;amp;amp;amp;quot;/echo/json/&amp;amp;amp;amp;amp;quot;,
allowClear: true,
dataType: 'json',
delay: 250,
params: {
contentType: &amp;amp;amp;amp;amp;quot;application/json&amp;amp;amp;amp;amp;quot;
},
data: function(term, page) {
//Code for dummy ajax response
return {
json: complex_employee_response,
delay: 0
};
},
results: function(data, page) {
return {
results: data
};
},
cache: false
},
formatResult: function(i) {
return ' &amp;amp;amp;amp;amp;lt; div &amp;amp;amp;amp;amp;gt; ' + i.name + ' (' + i.role + ')
' + ' &amp;amp;amp;amp;amp;lt; /div&amp;amp;amp;amp;amp;gt;
';
}, // Formats results in drop down
formatSelection: function(i) {
return ' &amp;amp;amp;amp;amp;lt; div &amp;amp;amp;amp;amp;gt; ' + i.name + ' (' + i.role + ')
' + ' &amp;amp;amp;amp;amp;lt; /div&amp;amp;amp;amp;amp;gt;
';
}, //Formats result that is selected
dropdownCssClass: &amp;amp;amp;amp;amp;quot;bigdrop&amp;amp;amp;amp;amp;quot;, // apply css that makes the dropdown taller
escapeMarkup: function(m) {
return m;
} // we do not want to escape markup since we are displaying html in results
})
$('#preselectObjectDataButton').on('click', function() {
var _array = []
var o = new Object;
o.id = &amp;amp;amp;amp;amp;quot;1&amp;amp;amp;amp;amp;quot;;
o.name = &amp;amp;amp;amp;amp;quot;Srinivas Chekuri&amp;amp;amp;amp;amp;quot;;
o.role = &amp;amp;amp;amp;amp;quot;Architect&amp;amp;amp;amp;amp;quot;;
_array.push(o);
$('#select2_ajax_complex_id').select2('data', _array);
});